Modern web applications require more efficient solutions from developers. The days when the main functionality of the website was to display static text, handle clicks or send messages via a form are long gone. Very often, as developers, we face challenges related to user demands and growing data volume, which significantly affect website performance. Here reactive programming comes in handy – in the introduction to this programming approach, I will present its capabilities using the example of the RxJS library. Read the introduction to reactive functional programming and get to know the advantages and disadvantages of RxJS.
Go to:
- 1. Why use RxJS? Challenges for developers of modern web applications
- 2. Data Streams
- 3. What is RxJS observable?
- 4. Observable vs JavaScript promises
- 5. Hot vs cold observables
- 6. Creating observables and execution of observable
- 7. Operators
- 8. Subscriptions
- 9. Observer
- 10. RxJS pros
- 11. RxJS cons
- 12. Features of RxJS – the summary
Why use RxJS? Challenges for developers of modern web applications
When creating modern and efficient web applications, we are faced with numerous challenges. As developers, we need to bear in mind that, for example, we cannot block a user and make them wait until our website handles several events. So, what should we do if the application has to run an animation, load a loader, and react to the user’s action? How do you make it possible to handle all these situations at the same time? Here the paradigm of reactive programming with the RxJS library comes in handy.
Reactive programming is a paradigm for writing code based on asynchronous data streams.
It is a way of creating applications that respond to changes, as opposed to the typical, imperative way of programming, in which we explicitly make step-by-step instructions to handle these changes.
It sounds quite complicated as such an approach calls for a change in the way of thinking. However, thanks to certain solutions, you can smoothly switch to reactive programming – in the case of JavaScript, the RxJS library.

Data Streams
A stream is a sequence of data ordered over time. Data streams can be created from objects, variables, data structures, events (clicks), HTTP request responses, etc.
On its timeline, the stream can also emit an error (when an unexpected event occurs causing the stream to end) or earn completed status (which means the stream has emitted all the data). We can capture these methods (error and complete) to perform appropriate operations on them – you will find out more in the paragraphs below.
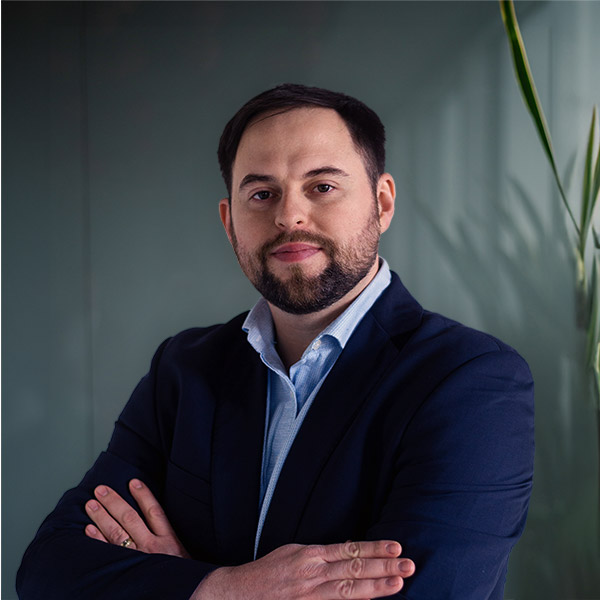
Elevate Your Application Development
Our tailored Application Development services meet your unique business needs. Consult with Marek Czachorowski, Head of Data and AI Solutions, for expert guidance.
Schedule a meetingWhat is RxJS observable?
RxJS uses observable objects that use an observer design pattern.
To illustrate how it works, let’s use the example of subscribing to a newsletter. You have likely clicked the “subscribe” button on YouTube or a blog many times already. When a new article or other material appears, you are notified straight away. Unsubscribing is possible at any time.
Subscribe
Observable and streams work the same way.
- By clicking the subscribe button, you become an observer of a given resource.
- Observable as an observed object gives us a data stream with news.
- The subscription to the stream provides us with the ‘subscribe’ method.
- We can also choose which data is valuable to us as ‘news’.
Observable vs JavaScript promises
Observable is a stream of values (of any type). But wait… Why do we need this observable stuff when we already have a promise object in JavaScript? Observable gives us a lot more possibilities. Here is a short comparison:
Promise
- it is asynchronous each time,
- it is ‘eager’ – processing starts immediately after an object is defined,
- it can only return one value,
- it has no operators,
- it cannot be canceled.
Observable
- it can be both synchronous and asynchronous,
- it is ‘lazy’ – this means that it won’t be executed at the moment of defining the stream, but when the subscription is created,
- it returns one or more values,
- there are a number of operators to use,
- we can cancel it any time,
- it gives us more options when handling errors (e.g. retry operator ()),
Hot vs cold observables
We can divide observables into hot and cold.
Cold observables:
- Emitting values begins with the first subscriber (when the first subscribe() appears – read more later in the article).
- They return new values for each new subscriber.
- Example: the service for downloading data from the backend, where we need to subscribe in order to execute the query.
Hot observables:
- They send values despite the lack of a subscriber.
- They share the same data among all subscribers.
- Example: observable created from a click event.
Creating observables and execution of observable
There are many ways to create an observable. Creating them from other data structures is also common – here of or from operators are helpful. In RxJS, creating one observable of several other observables is not a problem. Below are some examples:
Observable examples
The from operator can be iterated over only one argument (e.g. arrays, array-like elements – let’s take an array of objects as an example).
import { from, Observable } from 'rxjs'; const to ReadBooks = [ { bookId: 1, title: The psychology of money', author: 'Morgan Housel' publicationYear: 2020 }, { bookId: 2, title: 'The subtle art of not giving a f*ck', author: 'Mark Manson', publicationYear: 2016 }, { bookId: 3, title: How to talk to anyone', author: 'Leil Lowndes', publicationYear: 1999 }, { bookId: 3, title: 'Invent and wander', author: Jeff Bezos', publicationYear: 2020 }, ]; let source2$ = from(toReadBooks); source2$.subscribe(book => console.log(book.title));
This way, the array of objects has turned into an observable. Easy, isn’t it?
Operators
What if there are several streams and we need one observable? No problem! RxJS provides several operators to help us. One of them is a concat.
import { concat, from, Observable, of } from 'rxjs'; const toReadBooks = [ { bookId: 1, title: The psychology of money', author: 'Morgan Housel' publicationYear: 2020 }, { bookId: 2, title: 'The subtle art of not giving a f*ck', author: 'Mark Manson', publicationYear: 2016 }, { bookId: 3, title: How to talk to anyone', author: 'Leil Lowndes', publicationYear: 1999 }, { bookId: 3, title: 'Invent and wander', author: Jeff Bezos', publicationYear: 2020 }, ]; let source1$ = of('hello', 10, true, toReadBooks[0].title); source1$.subscribe(value => console.log(value)); let source2$ = from(toReadBooks); source2$.subscribe(book => console.log(book.title // combine the 2 sources concat(source1$, source2$) .subscribe(value => console.log(value));
Voilà! RxJS makes life easier!
Subscriptions
I mentioned the option of ‘subscribing’ to the stream. Another term that seems complicated at first (but isn’t!). A subscription simply means connecting to a stream. Each observable has a subscribe() method, to which we can pass parameters in two ways: as an object with methods or as a set of callbacks. The object passed to the subscribe method is called an observer.
Observer
The observer has three components:
- The first method (next) is executed if we manage to get the value from the stream. Each new value invokes this method.
- The second method (error) is executed if an error occurs in the stream, ; for example, in the HTTP query we get the status 500. In the event of an error, our observer will not proceed and will not perform the complete method, and thus will be marked as closed and will stop emitting values.
- The third method (complete) is executed when the stream emits the last value.

Nearshoring services
Entrust your project to nearshore software development experts Get started now!RxJS pros
The RxJS library undoubtedly facilitates application development. It can be integrated with practically any frontend framework (Angular, React). Also, using pure JavaScript is not a problem. There is a wide range of operators to use which allow you to modify streams in any way. Frequent updates make subsequent editions of the library easier to use. Thanks to RxJS, we can speed up, as well as improve the quality of software.
RxJS cons
I find it harder to write something about the disadvantages than the advantages. Working with Angular every day, I can’t imagine writing even one component without the RxJS library. Certainly, it might be difficult – especially for beginners – to test RxJS code. Testing such code calls for knowledge of many additional techniques and tools. Fortunately, RxJS has a solution for this in the form of RxJS Marbles. It allows us, step by step, to test asynchronous RxJS code synchronously, using the RxJS TestScheduler test tool and virtual time steps. However, this is an extensive issue that could better be described in a separate article.
Features of RxJS – the summary
The above is a very broad description of reactive programming and the RxJS library. A new article could be written on each of the above matters, and mine is just a drop in the ocean – many aspects have been skipped over.
Undoubtedly, RxJS has become standard for developing modern and efficient applications. Continuous development and support for the most popular frameworks make RxJS by far the most frequently used library, allowing us to control asynchrony.
Consult your project directly with a specialist
Book a meetingRead more: Using AWS Lambda functions
Go to:
- 1. Why use RxJS? Challenges for developers of modern web applications
- 2. Data Streams
- 3. What is RxJS observable?
- 4. Observable vs JavaScript promises
- 5. Hot vs cold observables
- 6. Creating observables and execution of observable
- 7. Operators
- 8. Subscriptions
- 9. Observer
- 10. RxJS pros
- 11. RxJS cons
- 12. Features of RxJS – the summary